Code snippets are small bits of code that can be added to enhance your website. You can insert widgets and script from source providers to your site. This will help the page include snippets which are most requested, and you can apply them site-wide by adding them to the header and footer portions of the website or on particular pages using the Code Snippet element.
You can copy and paste the code below to your website's header/footer code to add a top floating button. If you want it only on specific pages, then add it to page-specific header/footer code boxes.
- <style>
- .rounded-floating-btn {
- position: fixed;
- right: 20px;
- width: 50px !important;
- height: 75px !important;
- bottom: 20px;
- padding: 15px;
- z-index: 5;
- border-radius: 2px;
- cursor: pointer;
- background-color: #101010;
- box-shadow: 0 0 25px rgba(0, 0, 0, 0.3);
- color: #fff;
- font-size: 70%;
- font-weight: 400;
- }
- .floated-btn-svg-icon {
- width: 20px;
- fill: #fff;
- margin: 0 auto;
- margin-top: 3px;
- }
- .rounded-floating-btn .zptext {
- margin-top: 3px;
- }
- </style>
- <script>
- window.onload=function(){
- var div = document.createElement('div');
- div.innerHTML='<div class="zpelem-box zpelement zpbox-container zpdefault-section zpdefault-section-bg rounded-floating-btn" data-background-type="none" style="display:none" id="scrollToTop"><div class="floated-btn-svg-icon"><svg xmlns="http://www.w3.org/2000/svg" viewBox="0 0 268.831 268.832"><path d="M223.255 83.659l-80-79.998c-4.881-4.881-12.797-4.881-17.678 0l-80 80c-4.883 4.882-4.883 12.796 0 17.678 2.439 2.44 5.64 3.661 8.839 3.661s6.397-1.221 8.839-3.661l58.661-58.661v213.654c0 6.903 5.597 12.5 12.5 12.5 6.901 0 12.5-5.597 12.5-12.5V42.677l58.661 58.659c4.883 4.881 12.797 4.881 17.678 0 4.882-4.881 4.882-12.795 0-17.677z"></path></svg></div><div class="zptext zptext-align-center"><p>TOP</p></div></div>';
- div.addEventListener('click',function(e){
- e.preventDefault();
- window.scrollTo(0,0);
- })
- document.body.appendChild(div);
- }
- window.onscroll = function() {
- var threshold = 50; // Pixels after the scrol to top should show
- var scrollToTop = document.getElementById('scrollToTop')
- scrollToTop.style.display = (document.body.scrollTop > threshold || document.documentElement.scrollTop > threshold) ? 'block' : 'none';
- };
- </script>
How do I keep all accordions closed at start?
You can copy and paste the code below to your website's header/footer code to keep all the accordion folds closed at the start. If you want it only on specific pages, then add it to page-specific header/footer code boxes.
- <script>
- function closeAccordian(){
- var accordianHeader = $D.getByClass('zpaccordion-active')
- var accordianContent = $D.getByClass('zpaccordion-active-content')
- for(var i=0;accordianHeader.length>0;){
- $D.removeClass(accordianHeader[i],'zpaccordion-active')
- }
- for(var i=0;accordianContent.length>0;){
- $D.removeClass(accordianContent[i],'zpaccordion-active-content')
- }
- }
- document.addEventListener("DOMContentLoaded",closeAccordian)
- </script>
How do I keep all tabs closed at start?
You can copy and paste the code below to your website's header/footer code to keep all the tabs closed. If you want it only in specific pages, then add it to page-specific header/footer code boxes.
- <script>
- function closeTabs(){
- var tabHeader = $D.getByClass('zptab-active')
- var tabContent = $D.getByClass('zptab-active-content')
- for(var i=0;tabHeader.length>0;){
- $D.removeClass(tabHeader[i],'zptab-active')
- }
- for(var i=0;tabContent.length>0;){
- $D.removeClass(tabContent[i],'zptab-active-content')
- }
- }
- document.addEventListener("DOMContentLoaded",closeTabs)
- </script>
Copy and paste the CSS code below, under the Custom CSS section (Customization -> Code; Custom CSS Editor tab).
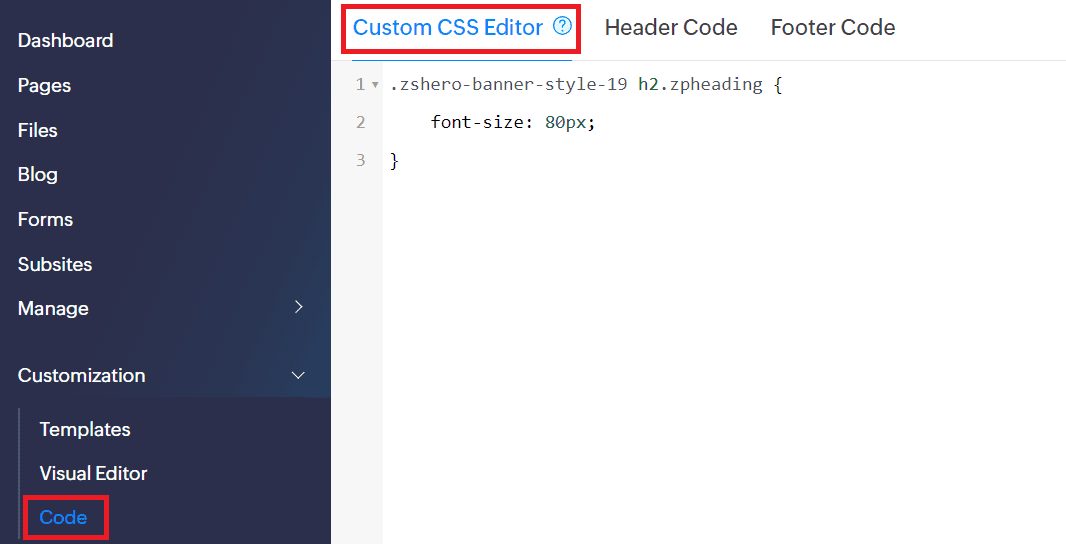
- .theme-blog-category-column .theme-blog-category-container.theme-blog-rss-feed {
- display: none;
- }
Copy and paste the code below to your website's header/footer code. If you want it only in specific pages, then add it to page-specific header/footer code boxes.
- <script type="text/javascript">
- var count = 0;
- var autoFillFunction = setInterval(
- function() {
- count++;
- var formElement = document.querySelector("[elname='<form_link_name>']");
- if(formElement != null) {
- var paramString = window.location.search.substr(1),
- paramArray = paramString.split("&");
- for(var i=0; i<paramArray.length; i++) {
- var fieldName = paramArray[i].split("=")[0];
- var fieldValue = paramArray[i].split("=")[1];
- var autoFillElement = formElement.querySelector("[name='" + fieldName +"']");
- autoFillElement.value = fieldValue;
- }
- clearInterval(autoFillFunction);
- } else if(count > 4) {
- clearInterval(autoFillFunction);
- }
- },2000);
- </script>
How do I list the latest blogs on any page?
Create a code snippet element in the place you want by dragging and dropping the Code Snippet.
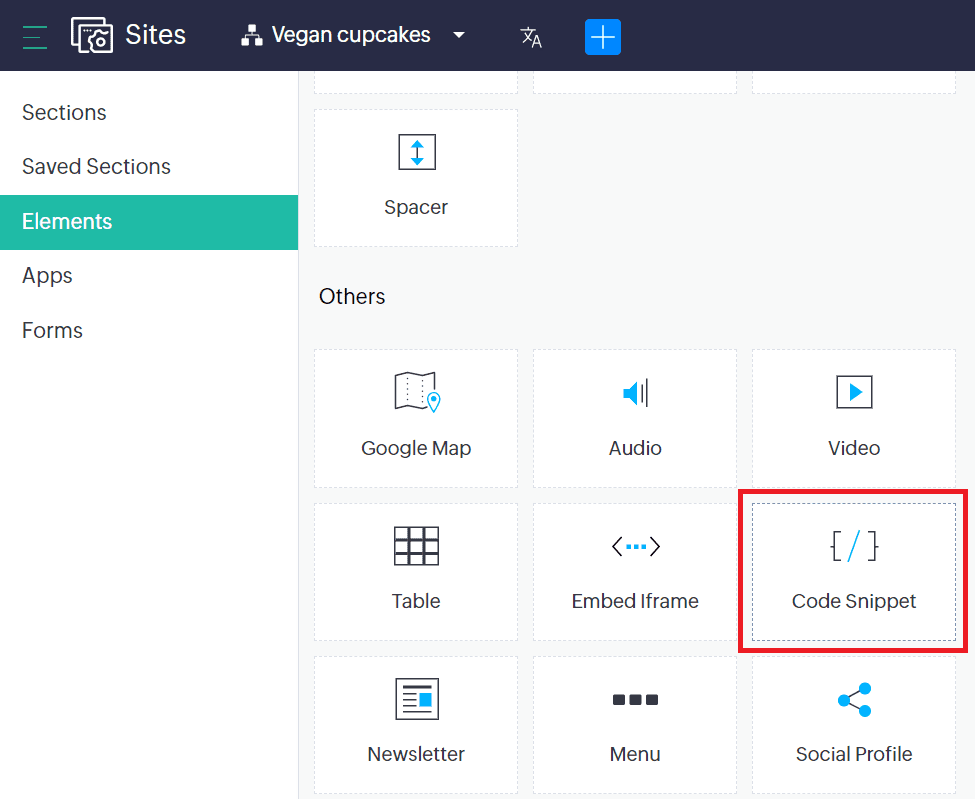
Now, paste the below code:
- <script src="https://cdnjs.cloudflare.com/ajax/libs/mustache.js/3.1.0/mustache.js"></script>
- <div id="template" style="display:none">
- <div data-element-type="row" class="zprow zpalign-items-flex-start zpjustify-content-flex-start ">
- {{#blog_list}}
- <div data-element-type="column" class="zpelem-col zpcol-md-4 zpcol-sm-12 zpalign-self- zpdefault-section zpdefault-section-bg ">
- <style type="text/css"></style>
- <div data-element-type="heading" class="zpelement zpelem-heading ">
- <style></style>
- <h3 class="zpheading zpheading-style-none zpheading-align-left " data-editor="true"><a href="{{link}}">{{title}}</a></h3>
- </div>
- <div data-element-type="text" class="zpelement zpelem-text ">
- <style></style>
- <div class="zptext zptext-align-left " data-editor="true">
- <p>{{{description}}}</p>
- </div>
- </div>
- <div data-element-type="button" class="zpelement zpelem-button ">
- <style></style>
- <div class="zpbutton-container zpbutton-align-left">
- <style type="text/css"></style><a class="zpbutton-wrapper zpbutton zpbutton-type-primary zpbutton-size-md zpbutton-style-none " href="{{link}}" target="_blank"><span class="zpbutton-content">Read More</span></a>
- </div>
- </div>
- </div>
- {{/blog_list}}
- </div>
- </div>
- <script>
- function renderList(list) {
- var template = document.getElementById('template').innerHTML;
- var rendered = Mustache.render(template, list);
- document.getElementById('list').innerHTML = rendered;
- }
- function getFeeds() {
- $X.get({
- url: '/blogs/feed',
- handler: function() {
- var result = this.responseText;
- if (window.DOMParser) {
- parser = new DOMParser();
- xmlDoc = parser.parseFromString(result, "text/xml");
- } else // Internet Explorer
- {
- xmlDoc = new ActiveXObject("Microsoft.XMLDOM");
- xmlDoc.async = false;
- xmlDoc.loadXML(result);
- }
- var items = xmlDoc.getElementsByTagName('item');
- renderList({ blog_list: itemsToJSON(items) })
- }
- });
- }
- function itemsToJSON(items) {
- var blog_list = [];
- for (var i = 0; i < items.length; i++) {
- var blog_post_json = {};
- var blog_post = items[i];
- var children = blog_post.children;
- for (var j = 0; j < children.length; j++) {
- var tagName = children[j].tagName;
- blog_post_json[tagName] = children[j].textContent;
- }
- blog_list.push(blog_post_json);
- }
- return blog_list;
- }
- document.addEventListener('DOMContentLoaded', getFeeds);
- </script>
- <div id="list">Loading...</div>
Zoho Flow Triggers - how to invite members to the member portal & create blog posts?
- Through Zoho Flow, Zoho Sites owners can trigger an invitation to the portal. The user can be assigned to a member portal group once he accepts the invitation and signs up.
Copy and paste the code below to your website's header/footer code. If you want it only in specific pages, then add it to the page-specific header/footer code boxes.
Step 1: Add this code to the header code of the website.
- <script>
- function openPopup(event, class_name) {
- event.preventDefault();
- var target = $D.getByClass(class_name)[0];
- $D.removeClass(target, 'zp-hidden-md');
- $D.removeClass(target, 'zp-hidden-sm');
- $D.removeClass(target, 'zp-hidden-xs');
- var modalContainer = $D.getByClass("modal-content")[0];
- modalContainer.appendChild(target);
- var closeElement = document.createElement('span')
- closeElement.classList += 'close'
- closeElement.innerHTML= '×'
- modalContainer.appendChild(closeElement)
- var modal = document.getElementById("myModal");
- modal.style.display = "flex";
- var span = document.getElementsByClassName("close")[0];
- if (span) {
- span.onclick = function() {
- modal.style.display = "none";
- }
- }
- window.onclick = function(event) {
- if (modal && event.target == modal) {
- modal.style.display = "none";
- }
- }
- setTimeout(function(){
- var from = $D.getByTag('from',div)[0];
- if(form) {
- $E.bind(form,'submit',ZPLPForm.IpFormSubmit);
- }
- }, 500);
- }
- </script>
Step 2: Add the code below in the footer code section of your site.
- <div id="myModal" class="modal"><div class="modal-content"></div></div>
Step 3: Add the code below to the custom CSS file.
- /* The Modal (background) */
- .modal {
- justify-content:center;
- display: none; /* Hidden by default */
- position: fixed; /* Stay in place */
- z-index: 300; /* Sit on top */
- padding-top: 100px; /* Location of the box */
- left: 0;
- top: 0;
- width: 100%; /* Full width */
- height: 100%; /* Full height */
- overflow: auto; /* Enable scroll if needed */
- background-color: rgb(0,0,0); /* Fallback color */
- background-color: rgba(0,0,0,0.4); /* Black w/ opacity */
- }
-
- /* Modal Content */
- .modal-content {
- display:inline-flex;
- position: relative;
- background-color: #fefefe;
- margin: auto;
- padding: 0;
- border: 1px solid #888;
- width: 60%;
- box-shadow: 0 4px 8px 0 rgba(0,0,0,0.2),0 6px 20px 0 rgba(0,0,0,0.19);
- -webkit-animation-name: animatetop;
- -webkit-animation-duration: 0.4s;
- animation-name: animatetop;
- animation-duration: 0.4s
- }
-
- /* Add Animation */
- @-webkit-keyframes animatetop {
- from {top:-300px; opacity:0}
- to {top:0; opacity:1}
- }
-
- @keyframes animatetop {
- from {top:-300px; opacity:0}
- to {top:0; opacity:1}
- }
-
- /* The Close Button */
- .close {
- color: white;
- position: absolute;
- top: -13px;
- right: -13px;
- float: right;
- font-size: 20px;
- font-weight: bold;
- border-radius: 50%;
- width: 30px;
- height: 30px;
- background:#969494;
- text-align:center;
- }
- .close:hover,
- .close:focus {
- text-decoration: none;
- cursor: pointer;
- }
-
- .modal-header {
- padding: 2px 16px;
- background-color: #5cb85c;
- color: white;
- }
-
- .modal-body {padding: 2px 16px;}
-
- .modal-footer {
- padding: 2px 16px;
- background-color: #5cb85c;
- color: white;
- }
Step 4: Add a section, click on the settings icon, and navigate to the visibility tab. Here, make visibility as hidden on all devices as below.
Step 5: Select the row inside the section that we made as hidden and select the CSS tab and input "target" in the class name, as shown below.
Step 6: Select the button and select JS tab, input openPopup(event,"target") in the function name input, with event type selected as onclick. Refer below:
Step 7: Publish the site, and on click of the button, row will open as popup like below. You can edit the row as popup requirement.

Note: The function used is openPopup(event,"target"). Here "target" is class name input in the element will be converted into a popup.