Keeping records on your customers and business prospects is essential for tracking data, conducting follow-ups, and running a business smoothly. When you use two separate applications, and store relevant data in each, checking and tracking data becomes more difficult.
Integrating applications that contain customer data is an effective solution for easily synchronizing and managing data across both applications. In this post, we'll look at how to integrate Zoho CRM with Zoho Workdrive in order to synchronize and manage Workdrive files and folders associated with a CRM contact across both applications.
Consider the following scenario: You've converted a lead to a contact in Zoho CRM. Now, you must associate files with that contact, such as legal documents or proofs. To do this, you can create a team folder where every team member can access and manage the contact's folders and files.
In order to store contact-specific files, you can create a new folder (within the team folder) for each WorkDrive contact by clicking a button within their CRM record. You can even create a widget for uploading files to the contact's Workdrive folder from within CRM.
Create a connector for Zoho Workdrive and add connector APIs
- Create a new connector in your Zoho Workdrive extension using the Connectors feature under Utilities in the left-side panel of the Zoho Developer console.
The connector details for the above example are as follows:
Request Token URL | https://accounts.zoho.com/oauth/v2/auth?scope=,WorkDrive.users.READ,WorkDrive.files.CREATE,
WorkDrive.teamfolders.CREATE&access_type=offline
|
Access Token URL | https://accounts.zoho.com/oauth/v2/token |
Refresh Token URL | https://accounts.zoho.com/oauth/v2/token |
Scopes | WorkDrive.users.READ,WorkDrive.files.CREATE,
WorkDrive.teamfolders.CREATE
|
The Zoho Workdrive REST APIs we added for our example are as follows:
Connector API Name | Method type | URL |
Get ZUID | GET | https://www.zohoapis.com/workdrive/api/v1/users/me |
Get all teams of a user | GET | https://www.zohoapis.com/workdrive/api/v1/users/${zuid}/teams |
Create team folder | POST | https://www.zohoapis.com/workdrive/api/v1/teamfolders |
Create new folder | POST | https://www.zohoapis.com/workdrive/api/v1/files |
File upload | POST | https://www.zohoapis.com/workdrive/api/v1/upload?filename=${file_name}&parent_id=${file_parent_id}&override-name-exist=true |
Create a settings widget to build team folder and assign them to Workdrive teams
- Create a settings widget to select a team to manage a contact's folders and files.
- We'll also create a CRM variable called "Team Folder ID" to store the assigned team's ID information for future operations.
Settings widget.js code snippet
Util={};
var teamidvalue;
//Subscribe to the EmbeddedApp onPageLoad event before initializing the widget
ZOHO.embeddedApp.on("PageLoad",function(data)
{
var data = {
}
//Invoking the 'Get ZUID' API to retrieve the user's ZUID
ZOHO.CRM.CONNECTOR.invokeAPI("xxx.workdrive.getzuid",data)
.then(function(dataa){
console.log(dataa);
response = dataa.response
responsejson=JSON.parse(response);
zuiddata=responsejson.data;
zuid=zuiddata.id;
var data = {
"zuid" : zuid
}
//Invoking the 'Get all teams of a user' API to fetch all the teams of a user
ZOHO.CRM.CONNECTOR.invokeAPI("xxx.workdrive.getallteamsofauser",data)
.then(function(dataa){
console.log(dataa);
response = dataa.response;
responsejson=JSON.parse(response);
teamdata=responsejson.data;
for (i = 0; i < teamdata.length; i++)
{
teamid=teamdata[i].id;
attributes=teamdata[i].attributes;
teamname=attributes.name;
var teamlist = document.getElementById("teamlist");
var option = document.createElement("OPTION");
option.innerHTML = teamname;
option.value = teamid;
teamlist.appendChild(option);
}
})
})
})
Util.getvalues=function()
{
//Retrieving the value chosen in the teamlist
teamidvalue=document.getElementById("teamlist").value;
/*Constructing data and passing to 'Create team folder' API to create a team folder called "CRM Contacts test"*/
var data = {
"extension_team_folder_name" : "CRM Contacts test",
"parent_id":teamidvalue
}
ZOHO.CRM.CONNECTOR.invokeAPI("xxx.workdrive.createteamfolder",data)
.then(function(dataa){
console.log(dataa);
response = dataa.response;
responsejson=JSON.parse(response);
teamfolderdata=responsejson.data;
teamfolderid=teamfolderdata.id;
//Set the ID of the team selected in the teamlist to the "Team Folder ID" CRM variable
var variableMap = { "apiname": "xxx__Team_Folder_ID", "value": teamfolderid};
ZOHO.CRM.CONNECTOR.invokeAPI("crm.set", variableMap);
ZOHO.CRM.API.getOrgVariable("xxx__Team_Folder_ID").then(function(data){
console.log(data);
});
});
}
|
Create a button in the Contacts module to make a new Workdrive folder for a contact
- Create a button called "Create a new workdrive Folder" using the Links & Buttons feature available in the Components section of the Zoho Developer console. Then, write a function to perform the desired action.
- For our use case, since we're creating a new folder specific to a contact inside a WorkDrive team folder, we'll create the folder with the Full Name of the Zoho CRM contact.
- We'll also create a custom field in the Contacts module called "Folder ID" to store the ID of the new Workdrive folder to perform future operations.
Create a new Workdrive folder: Function code snippet
//Fetching the current contact details and retrieving the Full Name and custom field folder ID of the contact
response = zoho.crm.getRecordById("Contacts",contact.get("Contacts.ID").toLong());
Fullname = response.get("Full_Name");
contactfolderid = response.get("xxx__Folder_ID");
if(contactfolderid == null)
{
/*Invoking the 'Create new folder' API to get create a folder in Workdrive for the Zoho CRM contact with the name as their Full Name*/
parentfolderid = zoho.crm.getOrgVariable("xxx__Team_Folder_ID");
dynamic_map = Map();
dynamic_map.put("name",Fullname);
dynamic_map.put("folder_parent_id",parentfolderid);
newfolderresp = zoho.crm.invokeConnector("xxx.workdrive.createnewfolder",dynamic_map);
newfolderresponse = newfolderresp.get("response");
newfolderdata = newfolderresponse.get("data");
newfolderid = newfolderdata.get("id");
contactinfo = {"xxx__Folder_ID":newfolderid};
/* Updating the 'Folder ID' custom field in contact's record with the new folder ID obtained from the response*/
folderresponse=zoho.crm.updateRecord("Contacts",contact.get("Contacts.ID").toLong(),contactinfo);
return "A new workdrive folder has been created with the ID - " + newfolderid;
}
else
{
return "Folder already present for contact in Workdrive";
}
|
- The above code snippet fetches the record details for the current contact to retrieve the customer's Full Name.
- The Workdrive parent folder ID (set using the settings widget), where the new folder for the contact will be created, is then retrieved using the getOrgVariable deluge task .
- The parent folder ID and the contact's Full Name are delivered to the Create new workdrive folder API to create a new folder for the contact in Workdrive.
- The ID of the new folder is then updated in the "Folder ID" custom field using updateRecord task.
Create a button in the Contacts module to upload files to a contact's Workdrive folder and associate it with a widget
- Create a button called "Upload file to Workdrive" using the Links & Buttons feature available in the Components section of the Zoho Developer console, then associate a widget to perform the desired action.
Upload file to workdrive - widget.html - Please find the attachment for the widget html code.
- The code snippet fetches the record details of the current contact, from which the custom field 'Folder ID' value is retrieved.
- The input file selected is also checked for its file type and name.
- The Folder ID and the file name are then constructed and passed as parameters to invoke the 'Upload File to Workdrive' API.
Sample output
- After installing the extension, authorize the Zoho Workdrive connector.
- Go to Settings on the extension configuration page.
- Choose a team to manage the contact's folders and files. Click Save.
- A new team folder, CRM Contacts test, is created in Zoho Workdrive for the chosen team.
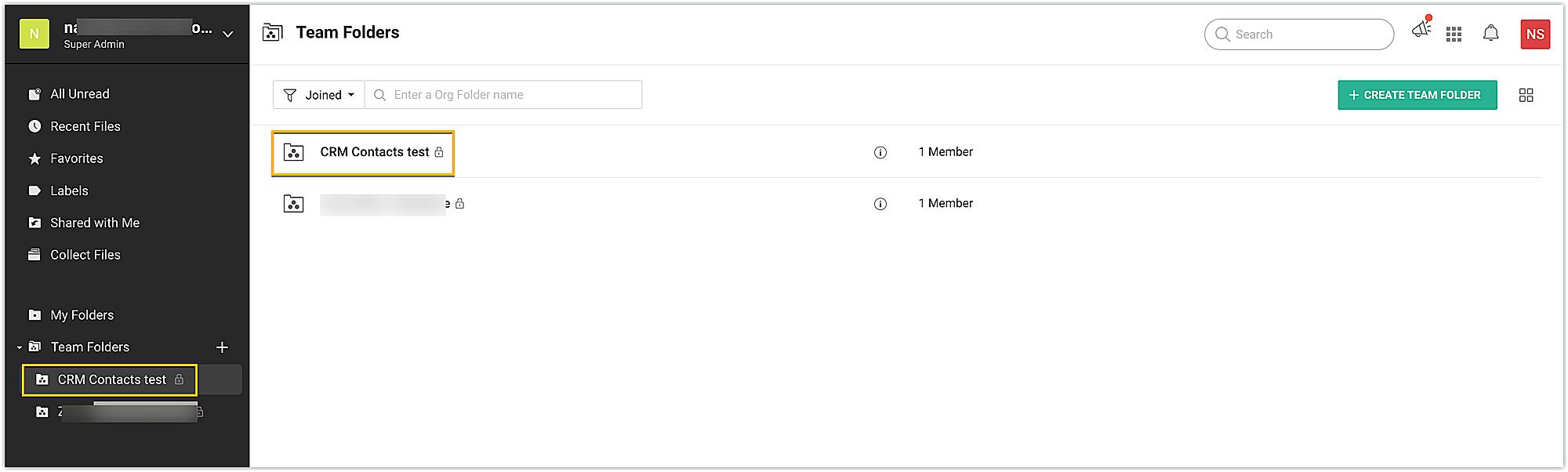
- Go to the Contacts module. Click on the Create a new workdrive folder button on the record's view page.
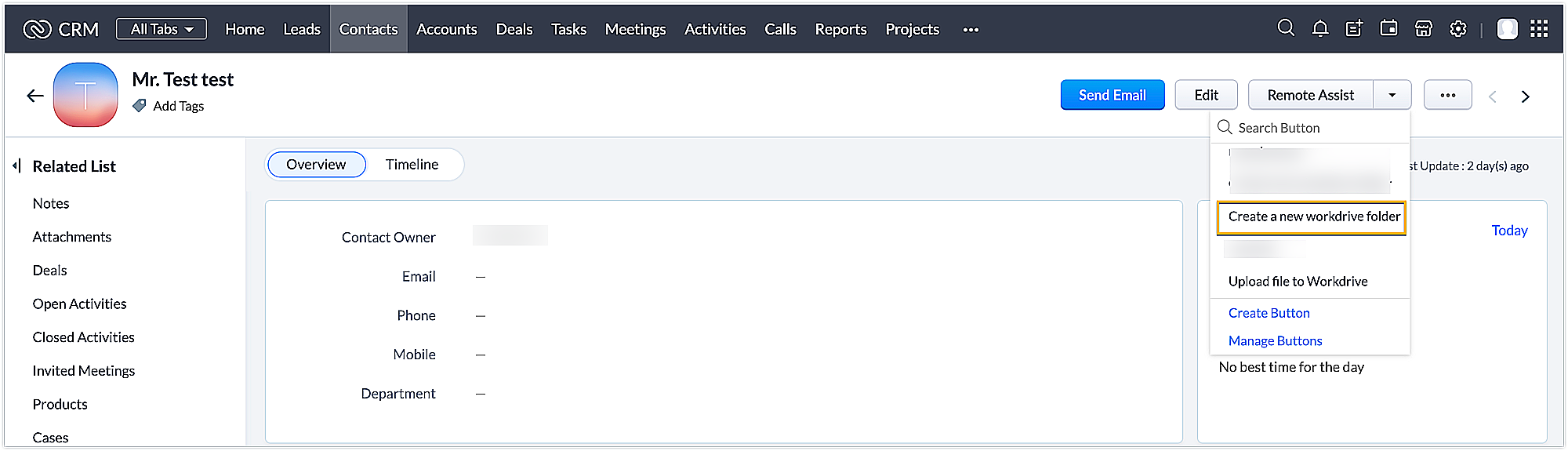
- A new folder is created in workdrive with the name of your contact.
- Once the folder is created, the custom field, Folder ID is also automatically updated with the new folder ID value.
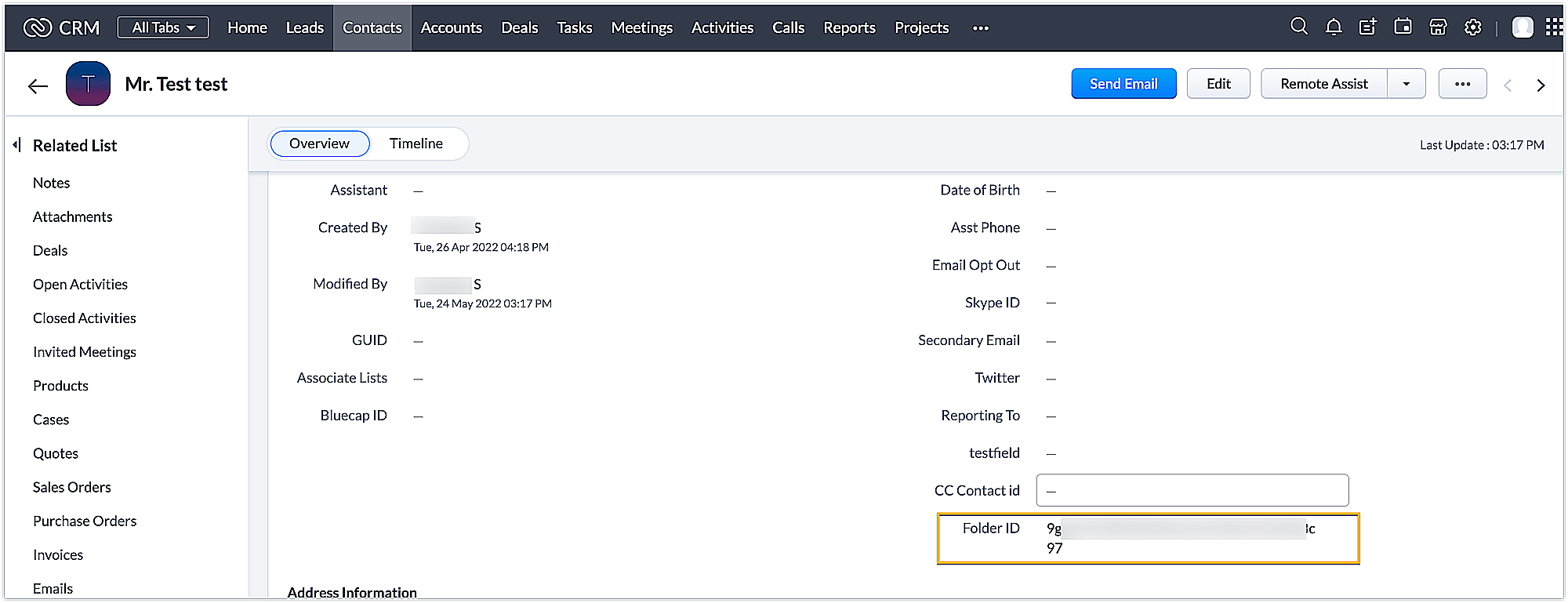
- Now, click on the Upload file to Workdrive button on the record's view page.
- The widget appears. Choose a file and click the Upload file to Workdrive button. The file will be uploaded to Workdrive.
Using this method, you can integrate Zoho CRM with Zoho Workdrive through an extension to perform necessaryfunctionalities for your business. We hope you find this information helpful!
In our next post, we will show you how to track, view, and access these Workdrive files within Zoho CRM. Keep following this space for more advice!
SEE ALSO
Recent Topics
Remove 30-Day Client Reply Restriction on Supervisor Rules in Zoho Desk
Dear Zoho Desk Team, I hope you're doing well. Currently, Supervisor Rules in Zoho Desk run once every hour but only apply to tickets that have received a customer response within the past 30 days. This restriction creates challenges for us, as we have
Paid Support Plans with Automated Billing
We (like many others, I'm sure) are designing or have paid support plans. Our design involves a given number of support hours in each plan. Here are my questions: 1) Are there any plans to add time-based plans in the Zoho Desk Support Plans feature? The
Contacts Don't Always Populate
I've noticed that some contacts can easily be added to an email when I type their name. Other times, a contact doesn't appear even though I KNOW it is in my contact list. It is possible the ones I loaded from a spreadsheet are not an issue and the ones
How to get NSE/BSE Stock Prices in Zoho sheets?
I've been looking for a function that provides me with the NSE/BSE listed stocks price in Zoho Sheets like GOOGLEFINANCE in Google sheets, but I found none. Please help if there is any way to het stock prices?
Zoho notes list issue
Hi team, Suddenly my extension is not working and I could not find the extension in my marketplace list anymore. Extension version deprecated This version of the extension is no longer in use. Please update the extension and try again.
Cannot edit email text in Zoho Form rules
I have a number of rules set up on a form depending on a user's submission. For some reason, I am no longer able to edit the content of the emails sent out based on those rules. I am invited to "use the advanced editor", but the original text of the email
How to Replace an Assessment in a Job Opening on Zoho Recruit
Hi everyone, I’m currently using Zoho Recruit and would like to replace the assessment linked to a specific job opening. I want to remove the existing assessment and add a new one. What is the best way to do this without losing any important data or affecting
Add "Lead Image" in Bulk?
Each of our Leads is accompanied with a URL containing a photo of the lead when they come in. We currently have to manually download then upload the photo to the lead. This is a HUGE waste of time. Is there any way to AUTOMATICALLY add the photos to the
Client script: Can not choose a date field for an onChange field event
Hi Zoho Team Why can't I choose one of my date fields to trigger an onChange event? Is this a client script limitation, or something wrong with my instance? If it is a limitation, is this mentioned in the documentation anywhere? Thanks. Marcus
Quickbooks conversion and Internet sales
Hello, we are considering converting from Quickbooks enterprise to Zoho Books and using MonotorZ for our mrp needs. Today we import Internet sales under a single customer with multiple ship to addresses. We want to maintain the detail in CRM for marketing
Can multiple agents be assigned to one ticket on purpose?
Is it possible to assign one ticket to two or more agents at a time? I would like the option to have multiple people working on one ticket so that the same ticket is viewable for those agents on their list of pending tickets. Is something like this currently
For security reasons your account has been blocked as you have exceeded the maximum number of requests per minute that can originate from one account.
Hello Zoho Even if we open 10-15 windows in still we are getting our accounts locked with error " For security reasons your account has been blocked as you have exceeded the maximum number of requests per minute that can originate from one account. "
how can I hide this Module?
Hi everyone, newbie question. how can I hide the "Sales Order" column? when I try I get this message: https://imghostr.com/86395c_p7j
UI Arabic
can i change the member portal UI to arabic in zoho community?
Domain verification is in progress... (How long do I need to wait?)
Trying to setup my first email domain by connecting with GoDaddy. Have been here for quite some time and the screen is not changing. How long should this take?Send DataSend Data
Custom Module missing SDK function fetchRelatedRecords(...) in a Client Script
Good day, We have added a new module with a Multi-Lookup relation to Contacts. When we tried to use the fetchRelatedRecords(id, related_list_api_name) function to get Related Records it is missing for our new custom module. https://js.zohocdn.com/crm/5124797/documentation/DotSDK/Modules.html
How to display profile picture for distribution list?
I am Admin of a Zoho Mail server and we have distribution lists along with user accounts. I am able to set Profile picture for the users and it shows when the email is sent to another companies. The members of the groups can also send email from those
Change email template depending on answer from form
Is it possible to set up the following in Zoho Desk: When a user submits a ticket via the Zoho Help Center's form, they can select an answer from a dropdown field. In this example, the dropdown options are 'Option A' and 'Option B.' If a user selects
Mail Search Not Working
Hello, Mail search is not working at all. I've tried Chrome and Mozilla. I can try and search for an exact term, or even an email that is 1st in my email list. All search does is sit and spin, or it comes up with no results. I've also tried it on my android
Password should not contain sequential characters
How can I avoid this? How do I even disable it. On my password policy page, it's all blank, so I don't know why I'm even getting this error now.
Same phone number for more than one account.
Hi there, I am a webdeveloper specialising in providing websites, webhosting and email solutions for my customers. I have signed up a number of my customers to Zoho Mail in the past, and a couple of these have grown into a paid package for Zoho CRM. As
Is there a live chat for Zoho mail?
I am having a problem in Zoho mail and would love to live chat with someone instead of email and wait for a response. Is there a function for this? I know there is in CRM but I can't seem to find it in mail... Thank you!
Integrating Zoho Desk Instances from two separate organizations
Is it possible to integrate Zoho Desk with an instance from another organization? For example, creating a ticket in one organization can cause the creation of a ticket in the second organization? Or certain tickets from one organization be viewable by
Why does incoming mail inconsistently bounce back from Zoho mail
On testing our user accounts, we are having problems where mail sent to zoho mail bounces back with errors message that 'relay access denied'. On testing from various accounts (including outlook, gmail and yahoo) mail seems to get through on some occasions
Zoho email setup in office365
When i am trying to setup zoho mail setup using my domain in office365 and it is not working and it says that we couldn't log on to the incoming (IMAP) server and please check your email address and password and try again. I was able to login using my
JunkMail rejected
Hello, we are facing problems sending mails. The IP has been blacklisted. Please, fix it as soon as possible. JunkMail rejected - sender4-op-o12.zoho.com [136.143.188.12]:17291 is in an RBL on rbl.websitewelcome.com, see Blocked - see http://www.spa
trying to access CRM Variables with JS SDK
Hello i built a widget with Sigma, i create CRM VARIABLES in custom properties. I try to access them in function : ZOHO.embeddedApp.on("PageLoad",function(data) with : ZOHO.CRM.CONFIG.getVariable("mycrmvariable").then(function(data){ console.log("mycrmvariable
My emails going to spam folder for hotmail or outlook
My emails (not spam mails) are going into the spam folder for my customers using hotmail. Gmail and Yahoo users are receiving the emails in their inbox. can you please solve this problem. I read few articles but coudnt find any solution to it. I am testing it by sending a simple text email no pictures nothing at all still it is filtering my emails as spam. Please help I am really loosing time and clients due to this. Thanks
Capture hotkeys inside the remote session and allow file exchange via clipboard
Hi guys, assist is a really good app, and to become great it would be nice to have some features other vendors have in place and we take them for granted. For example, ScreenConnect, TeamViewer and others allow you to send hotkeys via the remote connection,
Chat function not working properly
Ever since upgrading to plus, the chat is all messed up. it is coming up behind the web page so that I cannot see what I'm typing and cannot read replies. I can only see the bottom of the text box at the bottom of the page, and then it is blocked. I've
Self Client Authorization Issue
Hi. Trying to test the api integration for Zoho Desk with the Self Client - Client Credintials flow method. I've created the self client, obtained the client id and secret, inputted "Desk.tickets.ALL" as my scope, and "ZohoDesk.[My Zoho Desk Org ID]"
Mass pdfs into OCR field
I am working on a Creator app that my org will use internally. Is there any way to mass upload pfs through a form with an OCR file upload field? Is Creator capable of this, or would I need to use Catalyst?
Cannot fetch url with custom extension (sigma - javascript)
Hello i try to make my first extension with API request, i have two cases 1) this a deluge code attach to a button --> this one works very well response = invokeurl [ url :"my_api_fetch_url" type :GET headers:{"api_key":"myapikey","accept":"application/json","content-type":"application/json"}
Wrong Time on Exported Records
Hello, All records in my exported Notes .csv file have the incorrect time for Created Time. They are all 8 hours ahead. I've already verified that my time zone is correct in both Personal Settings and Company Settings. Is there any way to fix this?
how to upload the picture and document
i want to upload the picture and document,would you please told how to upload them?could you told it for each step?
Zia Call Intelligence only up 10 License
I have been trying to install Call Intelligence for two days now, but strangely, the button is missing at this point. The documentation could be better, but most importantly, someone should inform small businesses like us that they don’t even bother enabling
Forgot my email management account
Hello, I am the administrator of ihomemix.com. I can’t remember which email address I used to register the account and then opened the email service for ihomemix. I can’t see the subscription period of my email function when I log in with this account.
Add customer to account based on domain name.
I generate reports based on a the account field, i.e. companyX. In GoToAssist, my last provider, there was an option to automatically assign new ticket creators to a company (or account) based on their domain name. So for example, if a new employee creates a ticket from @companyx.com, for them to be automatically added to the companyx account would be a huge advantage. As it stands right now, I have to remember to add them to the account manually. Often I forget and when generating a report for
Facilitate business processes by mandating Kiosks in your Blueprint's transition settings
Hello everyone, We've made a few enhancements to Kiosk Studio. Blueprints provide a structured and systematic approach to executing business processes, and you can use Kiosks to build custom capabilities to retrieve, collect, and execute actions on CRM
Response Violation - Zoho Desk
Hi Team, I just need an information regarding the zoho desk - Response Violation and how can we avoid the tickets from getting the tickets response violated.
Next Page