Integrate ASAP SDK to implement the help center services within your Flutter applications.
ASAP is a self-service platform that provides the following help center services to the customers:
- Raise tickets
- Access the Knowledge Base
- Interact with other customers through community
- Enhance self-service with Zia Bot and Guided Conversations
Embedding ASAP add-on into your app
The ASAP SDK provides code snippets that allows you to effortlessly integrate help center components into your Flutter application. By utilizing ASAP SDK, developers can save time and effort by avoiding the need to build these features from scratch.
Embedding ASAP add-on into your app using the Flutter SDK includes the following steps:
Step 1: Create a mobile add-on in Zoho Desk
To create the add-on, perform the following steps:
In Zoho Desk, go to Setup > Channels > ASAP.
In the Setup pane on the left, under ASAP, click the + button next to Mobile. The Create Mobile Add-On page appears.
Under Add-On Details, configure the following settings:
Name: Name for the ASAP add-on. The name is only for reference; it does not appear anywhere on the app UI.
Available for: Departments for which this ASAP add-on must be enabled.
Bundle ID: Enter the bundle ID of your app in the appropriate field for iOS/Android.

Bundle ID is mandatory for enabling Live Chat and Push Notifications.
Chat: Toggle switch to enable or disable the Chat feature, offering three options for customization. You can choose to enable or disable Zia, Guided Conversations(GC), and Live Chat.
The Live Chat component reflects the settings configured for the chat channel in your help desk portal. Therefore, any change required must be done via the Setup page in Zoho Desk.Push Notifications: Toggle switch to enable or disable Push Notifications.
JWT-based user authentication is mandatory for enabling Ticket notifications. Push Notifications related to tickets are not sent to anonymous users.
Authentication Method: User authentication setting for the add-on
Anonymous (guest) users : The end-users who are not authenticated are anonymous users. They can only submit tickets, view posts in the user community, and chat with a customer support agent. They cannot view the tickets they submitted or actively participate in the user community.
JWT (registered) users : The registered end-users are authenticated users. The JWT authentication method is used to verify the end user's identity. In addition to the activities that guest users can perform, authenticated users can also view and track the status of the tickets they submitted, reply/comment on a ticket, and actively participate in the user community (with rights to perform actions such as following a topic, adding a topic, and adding a comment to existing posts).
4. Click Save.
A new section called Code Snippet appears. This section displays the Organization ID, App ID, and Datacenter details of your app. These details are vital for initializing the ASAP SDK in your app.
Step 2: Install the plugin
To install the plugin in, run either of the following commands:
flutter pub add zohodesk_portal_services
(or)
add the following in pubspec.yaml
dependencies:
zohodesk_portal_services: version
Step 3: Resolve the ASAP SDK dependencies in your app
Android:
The dependencies of the ASAP SDK are available at maven.zohodl.com. To include the dependencies in your Android project, you must add the relevant Maven URLs to your project's build.gradle file.
To perform this, open the Android folder of your Flutter project in Android Studio and add the following Maven repository in the project level build.gradle file.
allprojects
{
repositories
{
maven { url 'https://maven.zohodl.com/'}
maven { url 'https://downloads.zohocdn.com/wmslibrary' }
}
}
iOS:
There is no need to separately import iOS-specific dependencies into the project since the iOS SDK dependencies are automatically added to Flutter projects through the native part of the Flutter plugin.
Step 4: Initialize the SDK
The following three keys are essential to initialize the SDK in your app:
- Organization ID(orgId)
- App ID(appId)
- Datacenter (dc)
To initialize the SDK, include the following code snippet in the initState() method of the main.dart file:
import 'package:zohodesk_portal_apikit/zohodesk_portal_apikit.dart' show ZohodeskPortalApikit;
ZohodeskPortalApikit.initializeSDK(orgId, appId, dc);
The values of the keys, orgId, appId, and dc appear under the Code Snippet section in the setup page of the ASAP add-on in Zoho Desk.

Code is common for both iOS and Android platforms.

Datacenter values according to the deployment type are as follows:
CN - DataCenter.CN
EU - DataCenter.EU
US - DataCenter.US
IN - DataCenter.IN
AU - DataCenter.AU
JP - DataCenter.JP
Configuring the UI
The configuration of the help center components is based on your Zoho Desk Help Center settings. To display the available components in your application, you need to include the relevant code snippets.
(i) Home
To display the ASAP SDK Home screen, use the following code snippet:
import 'package:zohodesk_portal_core/zohodesk_portal_core.dart' show ZohodeskPortalCore;
ZohodeskPortalCore.showHome();
(ii) Knowledge Base
To display the Knowledge Base screen, use the following code snippet:
import 'package:zohodesk_portal_kb/zohodesk_portal_kb.dart' show ZohodeskPortalKb;
ZohodeskPortalKb.show();
To display the Community screen, use the following code snippet:
import 'package:zohodesk_portal_community/zohodesk_portal_community.dart' show ZohodeskPortalCommunity;
ZohodeskPortalCommunity.show();
To display the Add Topic screen, use the following code snippet:
import 'package:zohodesk_portal_community/zohodesk_portal_community.dart' show ZohodeskPortalCommunity;
ZohodeskPortalCommunity.showTopicForm();
(iv) Tickets
To display the Tickets List screen, use the following code snippet:
import 'package:zohodesk_portal_ticket/zohodesk_portal_ticket.dart' show ZohodeskPortalTicket;
ZohodeskPortalTicket.show();
To display the Add Ticket screen, use the following code snippet:
import 'package:zohodesk_portal_ticket/zohodesk_portal_ticket.dart' show ZohodeskPortalSubmitTicket;
ZohodeskPortalSubmitTicket.show();
(v) Live Chat
To display the Live Chat screen, use the following code snippet:
import 'package:zohodesk_portal_chat/zohodesk_portal_chat.dart' show ZDPortalChat;
ZDPortalChat.show();
(vi) Guided Conversations(GC) and Zia
To display GC or Zia, use the following code snippet:
import 'package:zohodesk_portal_gc/zohodesk_portal_gc.dart' show ZDPortalGC;
ZDPortalGC.show();

Code is common for both iOS and Android platforms.
Customizing themes and theme type
Predefined themes
The SDK UI comes with two predefined themes: light and dark.
Android
iOS
Setting up a theme type
You can customize the theme type in the SDK add-on using the below code:
import 'package:zohodesk_portal_configuration/zohodesk_portal_configuration.dart'
show ZohodeskPortalConfiguration;
import 'package:zohodesk_portal_configuration/data/configuration_model.dart';
ZohodeskPortalConfiguration.setTheme(ZDPTheme.system);
ZDPTheme.system: By default, the system theme is selected. If you want to change the theme to light/dark mode, use the following methods:
- ZDPTheme.light : Changes the SDK add-on theme to light irrespective of the system theme.
- ZDPTheme.dark: Changes the SDK add-on theme to dark irrespective of the system theme.

Code is common for both iOS and Android platforms.
Customize colors in the particular theme
Android
To customize the colors of the particular theme, follow the below steps:
Step 1: To customize a particular color, include the following method in Android project folder Application class:
ZDPTheme theme = new ZDPTheme.Builder(true)
.setColorPrimary(Color.parseColor(colorString))
.setColorAccent(Color.parseColor(colorString))
.build();
ZDPortalConfiguration.setThemeBuilder(theme);
Step 2: Configure the theme type(dark or light) to reflect the customized color within the application as instructed in the
Setting up a theme type.
iOS
You can override the default dark and light theme in the SDK add-on
To update the default light theme, include the following method:
ZDPThemeManager.updateLightTheme(theme: <#ZDPThemeProtocol#>)
To update the default dark theme, include the following method:
ZDPThemeManager.updateDarkTheme(theme: <#ZDPThemeProtocol#>)
Here, ZDPThemeProtocol contains the list of properties that represents the most used colors across the SDK, such as primaryTextColor and navigationBarTextColor. All properties in the protocol are optional. You can create any class conforming to this protocol, implement one or more properties, and pass an object of that class to the updateLightTheme or updateDarkTheme method. The colors you set in the class will override the default theme.
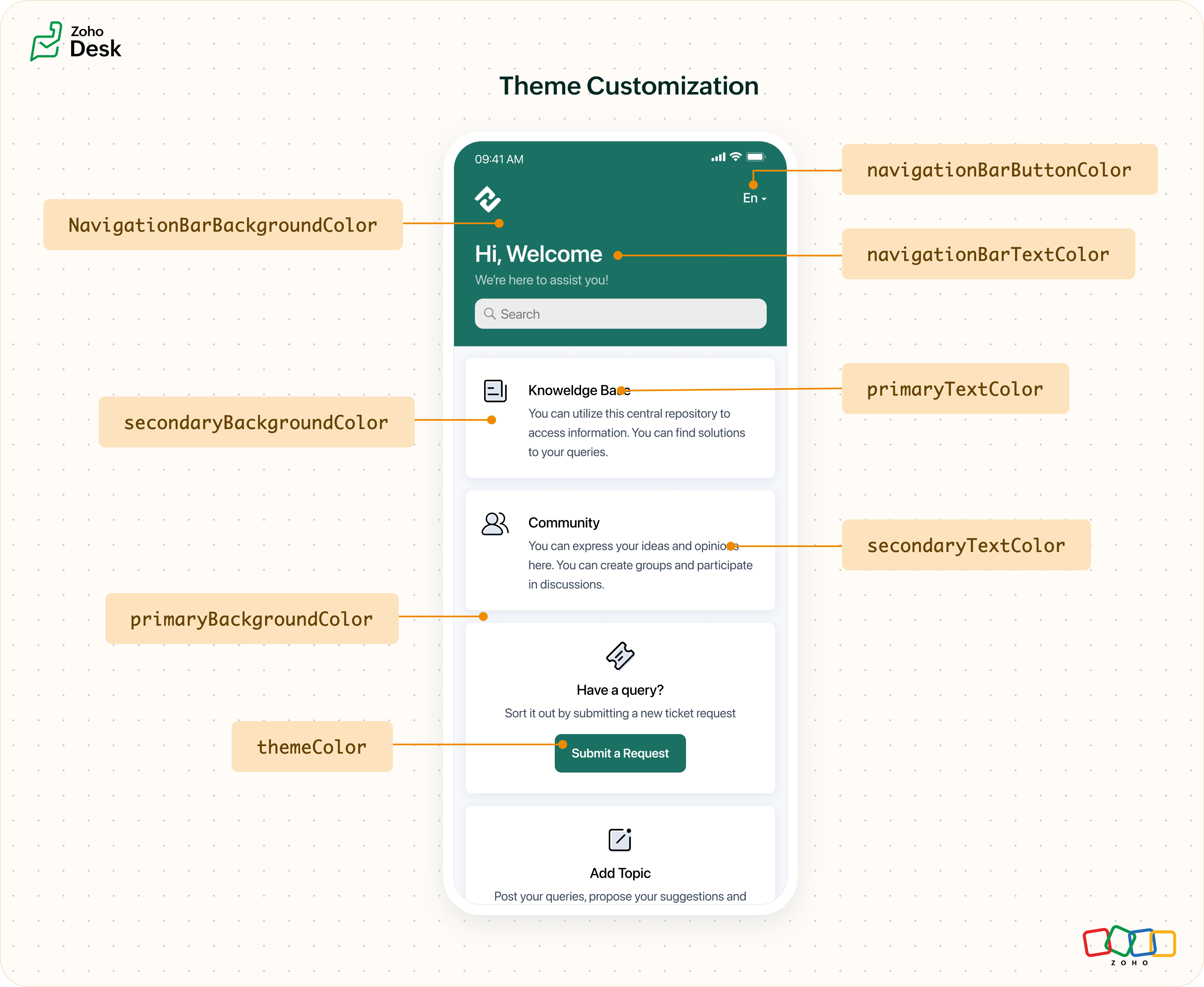

Setting up a theme type involves choosing a predefined theme type. The SDK's theme can be customized, but its overall appearance will always be either dark or light, depending on the theme type you have chosen.
Configuring user authentication in the add-on
(i) Authenticating users:
To authenticate users in the add-on, include the following code snippet:
import 'package:zohodesk_portal_apikit/zohodesk_portal_apikit.dart' show ZohodeskPortalApikit;
ZohodeskPortalApikit.login(jwtToken, (isSuccess)
{
//Sign in success/failed
});
In the above code snippet,
jwtToken refers to the token that is passed from your app. Read more on
how to generate jwttoken- callback will be called after userFetch is executed.
- isSuccess will indicate whether the user authentication was successful or not.
(ii) Logging out users:
To log users out from the add-on, include the following code snippet:
import 'package:zohodesk_portal_apikit/zohodesk_portal_apikit.dart' show ZohodeskPortalApikit;
ZohodeskPortalApikit.logout((isSuccess)
{
//Sign out success/failed
});
- callback will be called after userFetch is executed.
- isSuccess will indicate whether the user's logout attempt was successful or not.

Code is common for both iOS and Android platforms.
Push Notifications
Push notifications are sent to the end-users on the following situations:
- Ticket priority update
- Ticket status update
- Agent's reply
- Agent's public comment
- Agent's reply to the Live Chat
Configuring Push Notifications settings
Ensure that the Push Notifications setting is enabled on the ASAP setup page in Zoho Desk. For more information, refer to '
Push Notifications' section under "
Create a mobile add-on in Zoho Desk."
For iOS, create and upload a valid Apple Push Notification Service (APNs) certificate and its password.
Refer to document to learn about Apple Push Notifications.Enable Push Notifications
Android
To enable Push Notifications, include the following code snippet in the main.dart class file:
import package:zohodesk_portal_apikit/zohodesk_portal_apikit.dart show ZohodeskPortalApikit;
ZohodeskPortalApikit.enablePush(_fcmToken);
iOS
To enable push notifications, include the following method in the iOS application AppDelegate file.
func application
(_ application: UIApplication,
didRegisterForRemoteNotificationsWithDeviceToken deviceToken: Data)
{
let token = deviceToken.reduce
("", {$0 + String(format: "%02X", $1)}).uppercased()
ZohoDeskPortalSDK.enablePushNotification
(deviceToken:token, mode: .production)
}

To ensure the accuracy of notifications:
- Test the functionality in development mode.
- Transition to development mode by replacing ".production" with ".sandbox" in the provided code snippet and Desk Setup.
- Remember to switch back to production mode before deploying the application for end-customer usage.
Disable Push Notifications
To disable Push Notifications, include the following code snippet in the main.dart class file:
import package:zohodesk_portal_apikit/zohodesk_portal_apikit.dart show ZohodeskPortalApikit;
ZohodeskPortalApikit.disablePush(_fcmToken);
_fcmToken is the string that is retrieved using 'FirebaseMessaging.instance.getToken().'
Code is common for both iOS and Android platforms.Handling Push Notifications
To navigate end-users to the respective screen, depending on the type of push notifications received.
Android
Type of Push Notification | End user navigation page |
Single notification | Ticket List |
Bulk notification | Ticket Details |
Chat notification | Live Chat |
Prerequisite: Ensure that the notification icon is set before handling Push Notifications. Passing the Push Notification icon:
To pass the icon, include the following code snippet:
ZohodeskPortalConfigurationPlugin.setNotificationIcon(drtawableRes);
drtawableRes refers to the drawable identifier of the icon.
Handling push notifications:
To handle push notifications, include the following code snippet in the
FirebaseMessaging.onMessage.listen and
_firebaseMessagingBackgroundHandler of
main.dart class. Learn more on
handling push notifications.ZohodeskPortalConfiguration.handleNotification(map);
map refers to the notification message data that is retrieved using, remotemessage.data

Ensure that the
SDK initialization process is completed before calling the
handleNotification function.
iOS
To handle the Push Notifications, include the following code snippet in the application:didReceiveRemoteNotification method in the AppDelegate file.
override func application(_ application: UIApplication, didReceiveRemoteNotification userInfo: [AnyHashable : Any])
{
ZDPortalConfiguration.processRemoteNotification(userInfo: userInfo)
}
Error logging
Enabling the console log allows you to track error messages, helping in the identification and resolution of errors that may arise during execution.
By default, logs are disabled for the SDK.
To enable the logs, include the following code snippet:
import 'package:zohodesk_portal_apikit/zohodesk_portal_apikit.dart' show ZohodeskPortalApikit;
ZohodeskPortalApikit.enableLogs();

Code is common for both iOS and Android platforms.

The logs should be disabled before releasing the build.
Release Notes
Version 1.0.2
- Integrated the latest version (beta version 14) of the Android SDK for Flutter.
Version 1.0.1
- Fixed the issue faced during SDK initialization.